In Magento 2, the efficient execution of tasks is paramount to the seamless functioning of an online store. Enter cron jobs, the unsung heroes behind scheduled tasks, periodic updates, and automated processes that keep your Magento 2 store running like a well-oiled machine. Let’s delve into the world of cron jobs in Magento 2, exploring their significance, configuration, and the pivotal role they play in ensuring optimal performance.
Table of Contents
Understanding Cron Jobs
At its core, a cron job is a scheduled task that is executed at predefined intervals by the cron daemon, a time-based job scheduler in Unix-like operating systems. In Magento 2, cron jobs facilitate the automation of various essential processes, including indexing, caching, and sending transactional emails. By executing these tasks in the background at specified intervals, cron alleviate the need for manual intervention, ensuring timely updates and streamlined operations.
Create a custom Cron Job
To create a job you will either need to create a complete new module or you can use an existing module. There are two files which are responsible for scheduling a cron. These are crontab.xml file and the file which you will define inside the crontab.xml file. This file mostly reside inside a cron directory of the module. However the crontab.xml is created inside etc directory. Learn how to create a new module in magento 2.
crontab.xml
The crontab.xml file in Magento 2 serves as the configuration file for defining cron jobs and their schedules. It provides a structured format for specifying the frequency, command, and other parameters associated with each cron job. With crontab.xml, the developer can easily manage and customize the cron schedule for various tasks such as indexing, caching, and sending emails. This XML-based configuration file streamlines the process of setting up cron jobs in Magento 2, ensuring timely execution of essential tasks and maintaining the optimal performance of the e-commerce platform.
An example of crontab.xml file
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:module:Magento_Cron:etc/crontab.xsd">
<group id="default">
<job name="log_cron_job" instance="Learningmagento\Cronjobs\Cron\Logger" method="execute">
<schedule>* * * * *</schedule>
</job>
</group>
</config>
Let us know what the above code will do. We have define a default group for our cron.
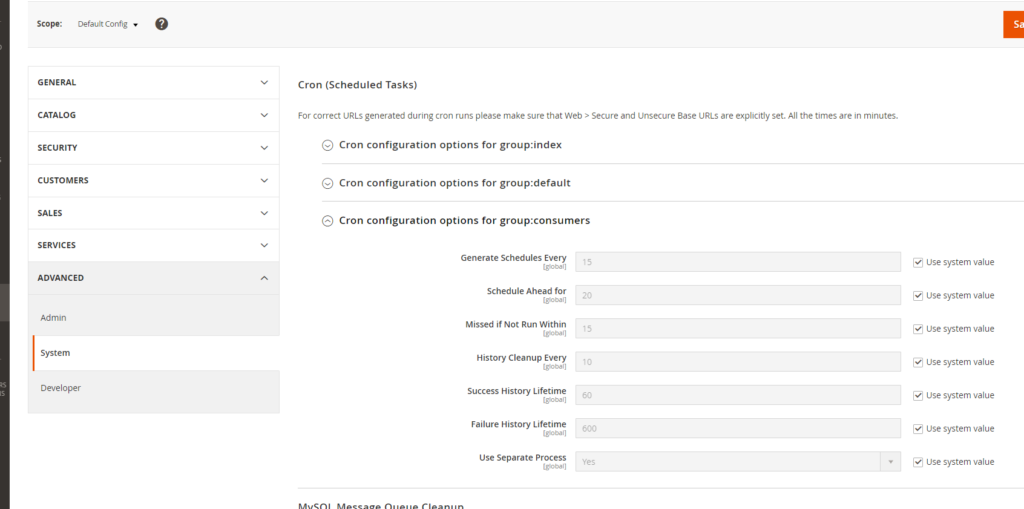
- group id:- The group id is the name of the cron group. There are different groups already present inside magento. Some of them are default, index and consumers. You can either use these groups or you can set up a custom cron group.
- job name:- This must be unique across all the cron job define. If same name exists then you will end up with an error inside the cron.log file.
- instance:- This include the path of the class which will be used when the cron is getting executed.
- method:- The method attribute contains the method of the class define in the instance path. This method has the code which is supposed to be ran when the cron is getting executed.
- schedule:- The element schedule contains the expression which is used by all the crons. You can design your own expression using the cron guru website. From the above example the cron will run every seconds.
Example of Instance Class
This class as mentioned above is supposed to be created under the cron directory. You can create the class anywhere an add the path in instance attribute. But it is recommend to keep the code clean and create this class under cron directory.
<?php
/**
*
* @category Custom Development
* @email contactus@learningmagento.com
* @author Learning Magento 2
* @website learningmagento.com
* @Date 01-04-2024
*/
namespace Learningmagento\Cronjobs\Cron;
class Logger
{
public function execute() {
$writer = new \Zend_Log_Writer_Stream(BP . '/var/log/logingcron.log');
$logger = new \Zend_Log();
$logger->addWriter($writer);
$logger->info('Testing cron');
}
}
After Development
After adding your code there are some thing which needed to be done otherwise it won’t work. You will first need to run the cache:clean command. The reason to run this command is because since you made a changes or have created a new xml file. The magento won’t see this changes unless you clear the cache.
Schedule cron from Configuration
Sometimes you need the cron to schedule by the Admin user of the magento in such case you can remove the schedule and place a configuration path. We will use the same example above.
crontab.xml
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:module:Magento_Cron:etc/crontab.xsd">
<group id="default">
<job name="log_cron_job" instance="Learningmagento\Cronjobs\Cron\Logger" method="execute">
<config_path>custom_cron_job/group_cron/schedule_expression</config_path>
</job>
</group>
</config>
system.xml
In the below file we have created a configuration for admin to set the cron scheduling. In the field we have define a source model class. In this class we will add option which the admin user can set.
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:module:Magento_Config:etc/system_file.xsd">
<system>
<tab id="learningmagento" translate="label" sortOrder="15">
<label>learning Magento</label>
</tab>
<section id="custom_cron_job" translate="label" sortOrder="20" showInDefault="1" showInWebsite="1" showInStore="1">
<label>Example of Cron Job</label>
<tab>learningmagento</tab>
<resource>Learningmagento_Cronjobs::default_config</resource>
<group id="group_cron" translate="label" type="text" sortOrder="10" showInDefault="1" showInWebsite="1" showInStore="1">
<label>Cron Group</label>
<field id="schedule_expression" translate="label" type="select" sortOrder="15" showInDefault="1" showInWebsite="0" showInStore="0">
<label>Schedule Expression</label>
<source_model>Learningmagento\Cronjobs\Model\Source\Config\Cron</source_model>
</field>
</group>
</section>
</system>
</config>
Model Class
<?php
/**
*
* @category Custom Development
* @email contactus@learningmagento.com
* @author Learning Magento 2
* @website learningmagento.com
* @Date 01-04-2024
*/
namespace Learningmagento\Cronjobs\Model\Source\Config;
use Magento\Eav\Model\Entity\Attribute\Source\AbstractSource;
/**
* Class Cron
* @package Learningmagento\Cronjobs\Model\Source\Config
*/class Cron extends AbstractSource
{
/**
* 1 mint
*/ const CRON_1MINUTE = '* * * * *';
/**
* 5 minis
*/ const CRON_5MINUTES = '*/5 * * * *';
/**
* 10 mins
*/ const CRON_10MINUTES = '*/10 * * * *';
/**
* 15 minus
*/ const CRON_15MINUTES = '*/15 * * * *';
/**
* 20 mins
*/ const CRON_20MINUTES = '*/20 * * * *';
/**
* 30 mins
*/ const CRON_HALFHOURLY = '*/30 * * * *';
/**
* hourly
*/ const CRON_HOURLY = '0 * * * *';
/**
* 2 hours each
*/ const CRON_2HOURLY = '0 */2 * * *';
/**
* daily once
*/ const CRON_DAILY = '0 0 * * *';
/**
* twice a day
*/ const CRON_TWICEDAILY = '0 0 12 * * *';
/**
* Get Cron timings list
*
* @return array
*/ public function getAllOptions()
{
$expressions = [
[
'label' => __('Every 1 Minute'),
'value' => self::CRON_1MINUTE
],
[
'label' => __('Every 5 Minutes'),
'value' => self::CRON_5MINUTES
],
[
'label' => __('Every 10 Minute'),
'value' => self::CRON_10MINUTES
],
[
'label' => __('Every 15 Minutes'),
'value' => self::CRON_15MINUTES
],
[
'label' => __('Every 20 Minutes'),
'value' => self::CRON_20MINUTES
],
[
'label' => __('Every Half Hour'),
'value' => self::CRON_HALFHOURLY
],
[
'label' => __('Every Hour'),
'value' => self::CRON_HOURLY
],
[
'label' => __('Every 2 Hours'),
'value' => self::CRON_2HOURLY
],
[
'label' => __('Once A Day'),
'value' => self::CRON_DAILY
],
[
'label' => __('Twice A Day'),
'value' => self::CRON_TWICEDAILY
],
];
return $expressions;
}
}
Essential Cron in Magento 2
Magento 2 relies on a set of core cron jobs to perform crucial tasks that are essential for the functioning of an online store. These tasks include indexing, cleaning the cache, generating sitemaps, and sending transactional emails. By scheduling these tasks to run at regular intervals, administrators can ensure that their Magento 2 store remains responsive, up-to-date, and capable of delivering an exceptional user experience to customers. You can also watch below video as he explains step by step process.
Troubleshooting Cron Job Issues
While cron jobs in Magento 2 are designed to operate seamlessly in the background, occasional issues may arise that require troubleshooting. Common issues include misconfigurations, insufficient server resources, or conflicts with other cron. To diagnose and resolve cron issues, administrators can leverage Magento’s built-in logging functionality, monitor server resources, and consult Magento’s official documentation for troubleshooting guidance. In some case a developer need to check table cron_schedule and see if some cron is halting the process.
Conclusion
In the dynamic realm of Magento 2 Cronjob serve as the backbone of automated task management, ensuring the smooth operation of online stores and empowering administrators to focus on strategic initiatives. By understanding the significance of Cronjob, configuring them effectively, and optimizing their performance, Magento 2 administrators can harness the full potential of this powerful toolset, driving efficiency, reliability, and scalability in their e-commerce endeavours.